Developer Pay Button Integration Manual Version 1.1
Button Payments allows you to connect via HTML and APIREST language to the functionality of the button.
For integration, the following processes are described:
- The following data must be sent through APIREST:
Type: GET | |
---|---|
Submit URL: https://portal.botonpagos.com/api/datafast/setOrder | |
1.1 Mandatory Parameters: | |
1.1.1 Establishment Code | code => text (30) (It is sent as the header of the request and this code is provided by Button Payments) |
1.1.2 Buyer’s First Name | first_name => text (30) |
1.1.4 Buyer’s last name | last_name => text (30) |
1.1.5 Buyer’s Identity Document | document => text (30) |
1.1.6 Buyer’s Phone | phone => text (100) |
1.1.7 Buyer’s Email | email => text (100) |
1.1.8 Purchaser’s address | address => text (200) |
1.1.9 Buyer’s IP Address | ip_address => text (30) |
1.1.10 Order ID | order_id => text (30) |
1.1.11 Order Total | total => double (10,2) |
1.1.12 Subtotal VAT 0 | subtotal0 => double (10,2) |
1.1.13 Subtotal VAT 12 | subtotal12 => double (10,2) |
1.1.14 Taxes | tax => double (10,2) |
1.1.15 Shipping cost excluding VAT | shipping => double (10,2) |
1.1.16 Shipping Tax Cost | shipping_tax => double(10,2) |
1.1.17 Products (Send in Json format) | products => array ( 0 => array ( id => text (30) nombre => text (100) isbn => text (45) (Optional) sku => text (45) (Optional) subtotal => double (10,2) tax => double (10,2) total => double (10,2) cantidad => int ) ) |
1.1.18 Order Status | status => text (30) Possible options: – on-hold – processing – completed – cancelled – pending |
1.1.19 Transaction Return Address (Only in web pages or systems, not valid for mobile app) | url_response => text (200) |
RECURRENCES | |
1.1.20 If the order is recurring | recurrent => boolean(or integer 1) |
1.1.21 Recurrence period in days | recurrent_period => int With the following table: 1 => Daily 2 => Weekly 3 => Biweekly 4 => Monthly 5=> Bimonthly 6 => Quarterly 7 => Biannual 8 => Annual |
1.1.22 Number of installments to be charged in the recurrence (optional) | recurrent_cuotas => int (0 is no limit) |
1.1.23 Specific collection day if the recurrence period is greater than 4 (Optional) | recurrent_day => int |
1.2 Optional Parameters: | |
1.2.1 Buyer’s Cell Phone | cellphone => text (100) |
1.2.2 Coupons (Send in Json format) | coupons => array ( [ code => text (30) (Coupon Code) amount => double (10,2) (Discount amount) ] ) |
<?php //I get the total values of the order //Subtotal 0 is the sum of the subtotals of the products that do not tax VAT $subtotal_order_0 = number_format(0, 2, '.', ''); //Subtotal 0 is the sum of the subtotals of the products that do tax VAT + the value of the Shipping (if applicable) $subtotal_order_12 = number_format(8.93, 2, '.', ''); //Tax is the value of the tax from the Subtotal $tax_order = number_format($subtotal_order_12*0.12, 2, '.', ''); //Total is the sum of the Subtotal + Tax $total_order = number_format($subtotal_order_0+$subtotal_order_12+$tax_order, 2, '.', ''); //I assemble the products of my order //Store Product ID $products[0]['id'] = 01; //Product name $products[0]['nombre'] = 'Nombre del Producto'; //Value without TAX of the product $products[0]['subtotal'] = 8.93; // Product tax $products[0]['tax'] = 1.07; //Total product value $products[0]['total'] = 10.00; //Product quantity $products[0]['cantidad'] = 1; //Store internal order id can be alphajumeric $order = "001"; //I assemble the array to send $datos = array( 'products' => json_encode($products), 'total' => $total_order, 'tax' => $tax_order, 'subtotal12' => $subtotal_order_12, 'subtotal0' => $subtotal_order_0, //Subtotal of products that do not tax VAT 'email' => "[email protected]", 'first_name' => "Primer Nombre Cliente", 'last_name' => "Apellidos Cliente", 'document' => "1717272354", //customer identity document 'phone' => "2238930",//Customer phone 'address' => "Dirección del cliente", 'ip_address' => get_client_ip(),//Function with client IP 'order_id' => $order, 'shipping' => 1.00, //Shipping value without taxes 'shipping_tax' => 0.12,//Value of the shipping tax, if there is a shipping value, the tax is mandatory by the Banks 'gateway' => 'botonpagos', 'status' => 'pending', 'date' => date('Y-m-d'), 'url_response' => 'https://prueba.com/resultado.php?order='.$order //This field is optional in the case of Mobile APPS ); //Address to ButtonPagos with the order details $url = 'https://portal.botonpagos.com/api/datafast/setOrder?'.http_build_query($datos); //Consultation via CURL $ch = curl_init(); curl_setopt($ch, CURLOPT_HTTPHEADER, array( 'code: ID del comercio'//ID provided by ButtonPagos )); curl_setopt($ch, CURLOPT_URL, $url); curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, true); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); $responseData = curl_exec($ch); //If there is a connection error if(curl_error($ch)){ echo curl_error($ch); } curl_close($ch); //Step to array the data obtained in Json $responseData = json_decode($responseData, true); /** * Function that allows to obtain the ip of the client making the payment * * */ function get_client_ip() { $ipaddress = ""; if (getenv("HTTP_CLIENT_IP")) $ipaddress = getenv("HTTP_CLIENT_IP"); else if(getenv("HTTP_X_FORWARDED_FOR")) $ipaddress = getenv("HTTP_X_FORWARDED_FOR"); else if(getenv("HTTP_X_FORWARDED")) $ipaddress = getenv("HTTP_X_FORWARDED"); else if(getenv("HTTP_FORWARDED_FOR")) $ipaddress = getenv("HTTP_FORWARDED_FOR"); else if(getenv("HTTP_FORWARDED")) $ipaddress = getenv("HTTP_FORWARDED"); else if(getenv("REMOTE_ADDR")) $ipaddress = getenv("REMOTE_ADDR"); else $ipaddress = "UNKNOWN"; return $ipaddress; } ?> <!doctype html> <html lang="en"> <head> <meta charset="utf-8"> <title>PayAgile</title> </head> <body> <div class="container"> <div class="row"> <div class="col-md-8 order-md-1"> <!-- I call the Payment Button on my page --> <iframe src="https://portal.botonpagos.com/api/datafast/botonV3/<?= $responseData['code'] ?>" width="100%" style="height: 766px; border: none;"></iframe> </div> </div> </div> </body> </html>
2. Create the Button Iframe
As a result of the previous step, a json object is obtained with an error code in case the sent data could not be processed. (Remember that to process like the example it is necessary to convert the json object into an array).The variable $responseData[‘error_code’] will be equal to 1 if there is an error and the description of the error will be contained in the variable $responseData[‘error_description’]
In case there is no error, the iframe can be implemented with the path + the code returned from the previous step and it is contained in the variable $responseData[‘code’]
The iframe would be as follows:
<iframe src=»https://portal.botonpagos.com/api/datafast/botonV3/<?= $responseData[‘code’] ?>» width=»100%» style=»height: 766px; border: none;»></iframe>
The result should be the following:
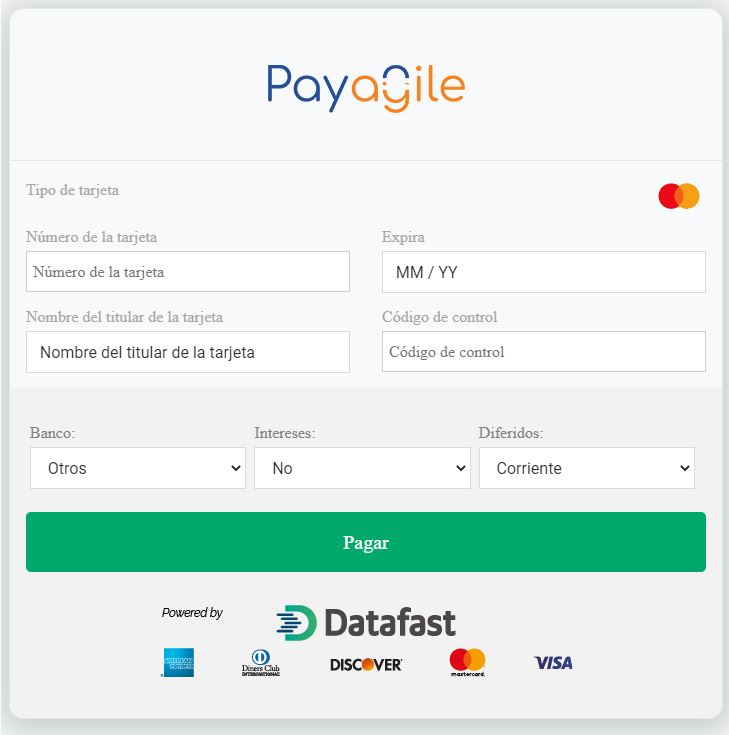
3. Once the client presses Pay, the page is redirected to the url_response sent in the first step
Then we proceed to call the following APIREST:
<?php $url = "https://portal.botonpagos.com/api/datafast/tienda/checkPayment/5eed292e12e42/".$_GET['order']."/".$_GET['id']; $ch = curl_init(); curl_setopt($ch, CURLOPT_URL, $url); curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, true); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); $responseData = curl_exec($ch); if(curl_error($ch)){ echo curl_error($ch); } curl_close($ch); $responseData = json_decode($responseData, true); ?> <!doctype html> <html lang="en"> <head> <meta charset="utf-8"> <title>PayAgile</title> </head> <body class="bg-light"> <div class="container"> <div class="row"> <div class="col-md-8 order-md-1"> <?php if($responseData ["error_code"]): ?> <div class="alert alert-warning" role="alert"> Payment error: <?= $responseData ["error_description"] ?> </div> <?php else: ?> <div class="alert alert-success" role="alert"> Payment completed successfully. An email was generated. </div> <?php endif; ?> </div> </div> </div> </body> </html>
Type: GET
Submit URL:
https://portal.botonpagos.com/api/datafast/tienda/getOrder/».$code.»/».$order
Where there are 2 variables:
Submit URL:
https://portal.botonpagos.com/api/datafast/tienda/getOrder/».$code.»/».$order
Where there are 2 variables:
- code => is the code of the establishment
- order => store order code (order_id sent in the first step)
$responseData [«error_code»] | => is 1 if there is an error in the transaction |
$responseData [«error_description»] | => error description |
$response [«id»] | => transaction id |
$response [«resultDetails»][«AuthCode»] | => authorization code |
$response [«resultDetails»][«ReferenceNbr»] | => reference number |
$response [«resultDetails»][«AcquirerResponse»] | => bank response |
response [«recurring»][«numberOfInstallments»] | => number of months deferred |
$response[«customParameters»][«SHOPPER_interes»] | => if it is with interest the operation |
$response[«customParameters»][«SHOPPER_gracia»] | => if you have months of grace |
$response[«paymentBrand»] | => card type |
$response[«card»][«bin»] | => banking entity |
$response[«card»][«binCountry»] | => country of the bank |
$response[«card»][«holder»] | => card owner |
$response[«cart»] | => product detail |
$response[«subtotal»] | => order subtotal |
$response[«tax»] | => tax |
$response[«total»] | => order total |